Connect with us!
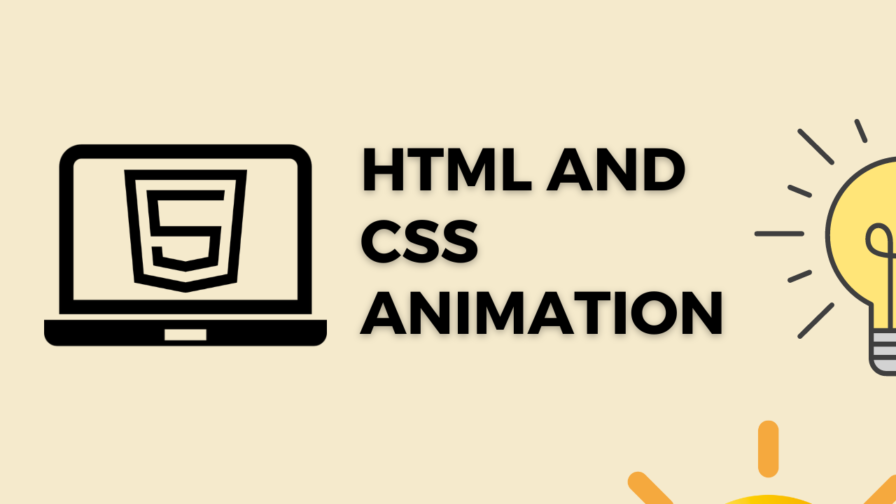
Blinking Dots Animation using CSS
In this article we will learn how to create Blinking Dots animation using CSS & HTML for your websites.
CSS Properties
Below mentioned are some of the important properties we use to create the circle intersect loading screen animation.
- Grid-area: The grid-area CSS shorthand property specifies a grid item’s size and location within a grid by contributing a line, a span, or nothing (automatic) to its grid placement, thereby specifying the edges of its grid area.
- Color: The color CSS property sets the foreground color value of an element’s text and text decorations, and sets the current color value. Thus current color may be used as an indirect value on other properties and is the default for other color properties, such as border-color.
- Margin-left: The margin-left CSS property sets the margin area on the left side of an element. A positive value places it farther from its neighbors, while a negative value places it closer.
- Transform-origin: The transform-origin CSS property sets the origin for an element’s transformations.
- Display: The display CSS property sets whether an element is treated as a block or inline element and the layout used for its children, such as flow layout, grid or flex.
- Animation-duration: The animation-duration CSS property sets the length of time that an animation takes to complete one cycle.
- Background: The background shorthand CSS property sets all background style properties at once, such as color, image, origin and size, or repeat method.
- Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.
- Content: The content CSS property replaces an element with a generated value. Objects inserted using the content property are anonymous replaced elements.
- Height: The height CSS property specifies the height of an element. By default, the property defines the height of the content area.
Blinking Dots Code Block
Here is the code for implementing the Blinking Dots Animation.
.star-hypno {
width: 100px;
height: calc(100px * 0.866);
margin-left: 150px;
color: #ffff00;
display: grid;
background: linear-gradient(
to bottom left,
#0000 calc(50% - 1px),
currentColor 0 calc(50% + 1px),
#0000 0
)
right/50% 100%,
linear-gradient(
to bottom right,
#0000 calc(50% - 1px),
currentColor 0 calc(50% + 1px),
#0000 0
)
left / 50% 100%,
linear-gradient(currentColor 0 0) bottom/100% 2px;
background-repeat: no-repeat;
transform-origin: 50% 66%;
}
.star-hypno::before,
.star-hypno::after {
content: "";
grid-area: 1/1;
background: inherit;
transform-origin: inherit;
}
.star-hypno::after {
animation-duration: 2s;
}
Yay! We have created the static Blinking Dots Animation.
Here is the output:
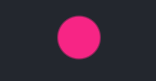
Blinking Dots CSS Classes
Below mentioned are the classes we use to create the Blinking dots loading screen animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes h5 {
100% {
transform: rotate(1turn);
}
}
The following way is to declare the CSS Class inside the class block.
.star-hypno {
width: 100px;
height: calc(100px * 0.866);
margin-left: 150px;
color: #ffff00;
display: grid;
background: linear-gradient(
to bottom left,
#0000 calc(50% - 1px),
currentColor 0 calc(50% + 1px),
#0000 0
)
right/50% 100%,
linear-gradient(
to bottom right,
#0000 calc(50% - 1px),
currentColor 0 calc(50% + 1px),
#0000 0
)
left / 50% 100%,
linear-gradient(currentColor 0 0) bottom/100% 2px;
background-repeat: no-repeat;
transform-origin: 50% 66%;
animation: h5 4s infinite linear;
}
.star-hypno::before,
.star-hypno::after {
content: "";
grid-area: 1/1;
background: inherit;
transform-origin: inherit;
animation: inherit;
}
.star-hypno::after {
animation-duration: 2s;
}
@keyframes h5 {
100% {
transform: rotate(1turn);
}
}
Following is the output for Blinking Dots Animation animation.
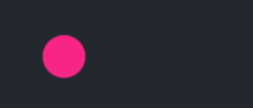
Video Tutorial
You can find the complete coding tutorial in the following YouTube Short video.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.star-hypno {
width: 100px;
height: calc(100px * 0.866);
margin-left: 150px;
color: #ffff00;
display: grid;
background: linear-gradient(
to bottom left,
#0000 calc(50% - 1px),
currentColor 0 calc(50% + 1px),
#0000 0
)
right/50% 100%,
linear-gradient(
to bottom right,
#0000 calc(50% - 1px),
currentColor 0 calc(50% + 1px),
#0000 0
)
left / 50% 100%,
linear-gradient(currentColor 0 0) bottom/100% 2px;
background-repeat: no-repeat;
transform-origin: 50% 66%;
animation: h5 4s infinite linear;
}
.star-hypno::before,
.star-hypno::after {
content: "";
grid-area: 1/1;
background: inherit;
transform-origin: inherit;
animation: inherit;
}
.star-hypno::after {
animation-duration: 2s;
}
@keyframes h5 {
100% {
transform: rotate(1turn);
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Star Hypno Animation - Salow Studios</h1>
<div class="star-hypno"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Blinking Dots Animation using CSS and HTML. See you in next tutorial!