Connect with us!
Playing Music Animation using HTML and CSS
A Playing Music Loader Animation, created using HTML and CSS, is a visually engaging element that simulates the appearance of a music player interface during the playback of audio content. Here’s a brief description of this type of animation:
You can find how to install and get started with Visual Studio code by checking out my tutorial – Running HTML using Live server in Visual Studio Code Editor – Salow Studios
Table of Contents
CSS Properties
Following are the CSS properties used:
Height: The height CSS property specifies the height of an element. By default, the property defines the height of the content area. If box-sizing is set to border-box, however, it instead determines the height of the border area.
Background-repeat: The background-repeat CSS property sets how background images are repeated. A background image can be repeated along the horizontal and vertical axes, or not repeated at all.
Margin: The margin CSS shorthand property sets the margin area on all four sides of an element.
Background-size: The background-size CSS property sets the size of the element’s background image. The image can be left to its natural size, stretched, or constrained to fit the available space.
Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.
Background: The background shorthand CSS property sets all background style properties at once, such as color, image, origin and size, or repeat method.
Code Block
Here is the code for implementing the Playing Music Animation.
.waveform {
margin: 70px;
width: 90px;
height: 120px;
--c: linear-gradient(#f72585 0 0);
background: var(--c) 0% 50%, var(--c) 50% 50%, var(--c) 100% 50%;
background-size: 18px 50%;
background-repeat: no-repeat;
}
Yay! We have created the static Playing Music Animation.
Here is the output:
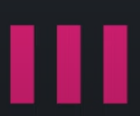
CSS Animation
pulsing animation can be implemented by scaling up and down the shape linearly.
Following are the classes used for animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes pulsing {
20% {
background-size: 18px 20%, 18px 50%, 18px 50%;
}
40% {
background-size: 18px 100%, 18px 20%, 18px 50%;
}
60% {
background-size: 18px 50%, 18px 100%, 18px 20%;
}
80% {
background-size: 18px 50%, 18px 50%, 18px 100%;
}
}
The following way is to declare the CSS Class inside the class block.
.waveform {
margin: 70px;
width: 90px;
height: 120px;
--c: linear-gradient(#f72585 0 0);
background: var(--c) 0% 50%, var(--c) 50% 50%, var(--c) 100% 50%;
background-size: 18px 50%;
background-repeat: no-repeat;
animation: pulsing 1s infinite linear alternate;
}
@keyframes pulsing {
20% {
background-size: 18px 20%, 18px 50%, 18px 50%;
}
40% {
background-size: 18px 100%, 18px 20%, 18px 50%;
}
60% {
background-size: 18px 50%, 18px 100%, 18px 20%;
}
80% {
background-size: 18px 50%, 18px 50%, 18px 100%;
}
}
Following is the output for Playing Music Animation animation.
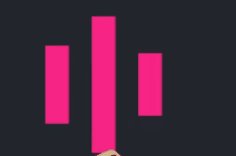
Video Tutorial
You can find the complete coding tutorial in the following YouTube Short video.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.waveform {
margin: 70px;
width: 90px;
height: 120px;
--c: linear-gradient(#f72585 0 0);
background: var(--c) 0% 50%, var(--c) 50% 50%, var(--c) 100% 50%;
background-size: 18px 50%;
background-repeat: no-repeat;
animation: pulsing 1s infinite linear alternate;
}
@keyframes pulsing {
20% {
background-size: 18px 20%, 18px 50%, 18px 50%;
}
40% {
background-size: 18px 100%, 18px 20%, 18px 50%;
}
60% {
background-size: 18px 50%, 18px 100%, 18px 20%;
}
80% {
background-size: 18px 50%, 18px 50%, 18px 100%;
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Playing Music Animation</h1>
<div class="waveform"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Playing Music Animation Animation using CSS and HTML. See you in next tutorial!