Connect with us!
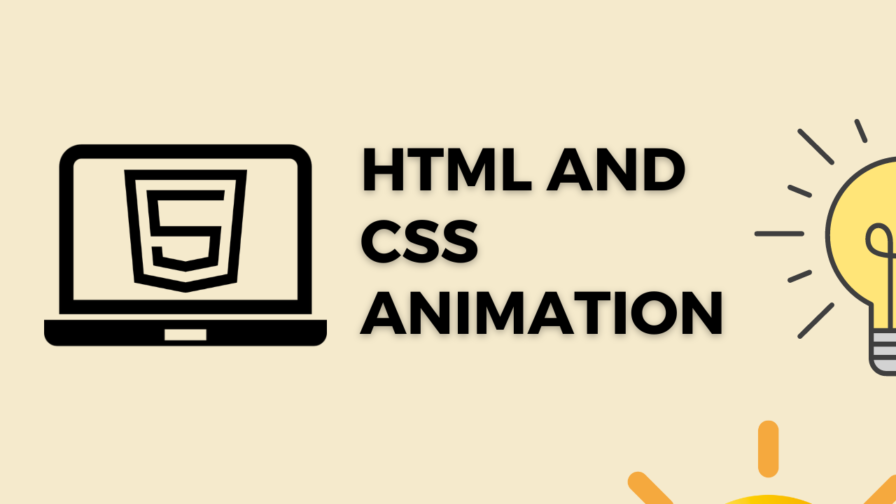
Circle Intersect Animation using HTML and CSS
CSS helps to create many interesting loading screen animations for creating your own website. This makes UI more appealing for the user.
CSS Properties
Below mentioned are some of the important properties we use to create the circle intersect loading screen animation.
- Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.
- Border-radius: The border-radius CSS property rounds the corners of an element’s outer border edge. You can set a single radius to make circular corners, or two radii to make elliptical corners.
- Grid-area: The grid-area CSS shorthand property specifies a grid item’s size and location within a grid by contributing a line, a span, or nothing (automatic) to its grid placement, thereby specifying the edges of its grid area.
- -webkit-mask: The mask CSS shorthand property hides an element (partially or fully) by masking or clipping the image at specific points.
- Mask-composite: The mask-composite CSS property represents a compositing operation used on the current mask layer with the mask layers below it.
- Content: The content CSS property replaces an element with a generated value. Objects inserted using the content property are anonymous replaced elements.
- Aspect-ratio: The aspect-ratio CSS property sets a preferred aspect ratio for the box, which will be used in the calculation of auto sizes and some other layout functions.
- Background: The background property sets all background style properties at once, such as color, image, origin and size, or repeat method.
- Mask: The mask CSS shorthand property hides an element (partially or fully) by masking or clipping the image at specific points.
- Display: The display CSS property sets whether an element is treated as a block or inline element and the layout used for its children, such as flow layout, grid or flex.
Circle Intersect Animation Code Block
Here is the code for implementing the Circle Intersect Animation.
.circle-anim {
width: 400px;
display: grid;
--mask: radial-gradient(
circle 12px at left 15px top 50%,
#0000 95%,
#000
),
radial-gradient(circle 12px at right 15px top 50%, #0000 95%, #000);
-webkit-mask: var(--mask);
mask: var(--mask);
-webkit-mask-composite: source-in;
mask-composite: intersect;
}
.circle-anim:before,
.circle-anim:after {
content: "";
grid-area: 1/1;
height: 30px;
aspect-ratio: 1;
background: #fff;
border-radius: 50%;
}
.circle-anim:after {
margin-left: auto;
}
Yay! We have created the static Circle Intersect Animation.
Here is the output:
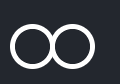
CSS Classes
Below mentioned are the classes we use to create the circle intersect loading screen animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS
@keyframes intersect {
to {
width: 40px;
}
}
Here we see how to declare the CSS Class inside the class block.
.circle-anim {
width: 400px;
display: grid;
--mask: radial-gradient(
circle 12px at left 15px top 50%,
#0000 95%,
#000
),
radial-gradient(circle 12px at right 15px top 50%, #0000 95%, #000);
-webkit-mask: var(--mask);
mask: var(--mask);
-webkit-mask-composite: source-in;
mask-composite: intersect;
animation: intersect 1s infinite alternate;
}
.circle-anim:before,
.circle-anim:after {
content: "";
grid-area: 1/1;
height: 30px;
aspect-ratio: 1;
background: #fff;
border-radius: 50%;
}
.circle-anim:after {
margin-left: auto;
}
@keyframes intersect {
to {
width: 40px;
}
}
And here is the final output.

Circle Intersect Animation Video Tutorial
I am attaching the video link for creating circle intersect animation which I have already posted in my YouTube channel. You can watch it if you are more interested.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.circle-anim {
width: 400px;
display: grid;
--mask: radial-gradient(
circle 12px at left 15px top 50%,
#0000 95%,
#000
),
radial-gradient(circle 12px at right 15px top 50%, #0000 95%, #000);
-webkit-mask: var(--mask);
mask: var(--mask);
-webkit-mask-composite: source-in;
mask-composite: intersect;
animation: intersect 1s infinite alternate;
}
.circle-anim:before,
.circle-anim:after {
content: "";
grid-area: 1/1;
height: 30px;
aspect-ratio: 1;
background: #fff;
border-radius: 50%;
}
.circle-anim:after {
margin-left: auto;
}
@keyframes intersect {
to {
width: 40px;
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Circle Intersect Animation</h1>
<div class="circle-anim"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Circle Intersect Animation Animation using CSS and HTML. See you in next tutorial!