Connect with us!
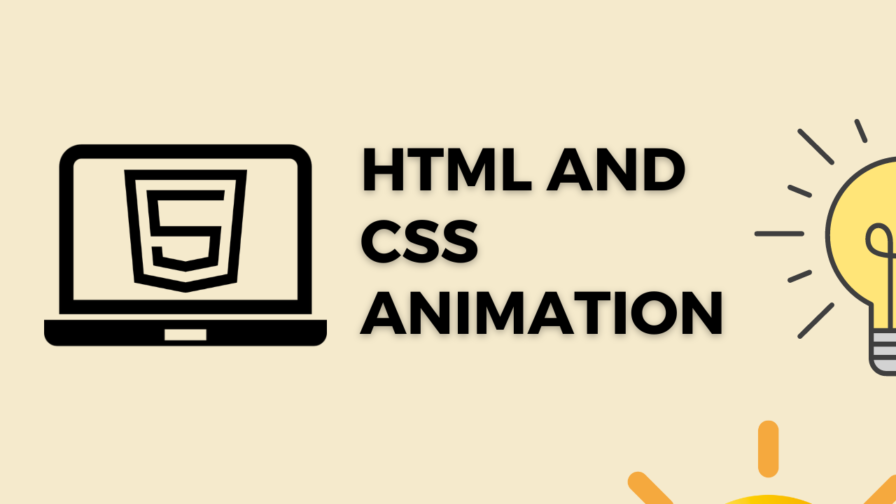
Convertible Triangle Animation HTML and CSS tutorial for beginners
To create a convertible triangle animation using html and CSS, you need to use the transform and transition properties. First, you need to create a div element with a class of con-triangle in your html file. Then, you need to style the div element with CSS to make it look like a triangle. You can use the border-width and border-color properties to create the triangle shape. Here is an example of the code:
Table of Contents
CSS Properties used for convertible triangle
Following are the CSS properties used:
Transform-origin: The transform-origin CSS property sets the origin for an element’s transformations.
Background: The background shorthand CSS property sets all background style properties at once, such as color, image, origin and size, or repeat method. Component properties not set in the background shorthand property value declaration are set to their default values.
Margin-left: The margin-left CSS property sets the margin area on the left side of an element. A positive value places it farther from its neighbors, while a negative value places it closer.
Display: The display CSS property sets whether an element is treated as a block or inline element and the layout used for its children, such as flow layout, grid or flex.
Content: The content CSS property replaces an element with a generated value. Objects inserted using the content property are anonymous replaced elements.
Animation-name: The animation-name CSS property specifies the names of one or more @keyframes at-rules that describe the animation to apply to an element. Multiple @keyframe at-rules are specified as a comma-separated list of names. If the specified name does not match any @keyframe at-rule, no properties are animated.
Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.
Clip-path: The clip-path CSS property creates a clipping region that sets what part of an element should be shown. Parts that are inside the region are shown, while those outside are hidden.
Height: The height CSS property specifies the height of an element. By default, the property defines the height of the content area. If box-sizing is set to border-box, however, it instead determines the height of the border area.
Code Block for convertible triangle
Here is the code for implementing the Convertable Triangle Animation.
.con-triangle {
display: flex;
margin-left: 120px;
width: 120px;
height: 120px;
}
.con-triangle:before,
.con-triangle:after {
content: "";
width: 50%;
background: #f72585;
clip-path: polygon(0 0, 100% 50%, 0% 100%);
animation-name: con-1;
transform-origin: bottom left;
}
.con-triangle:before {
clip-path: polygon(0 50%, 100% 0, 100% 100%);
transform-origin: bottom right;
--s: -1;
}
Yay! We have created the static Convertable Triangle Animation.
Here is the output:
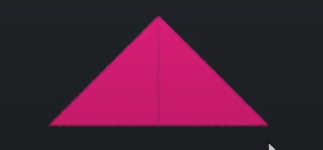
CSS Animation for convertible triangle
con-0 , con-1 animation can be implemented by scaling up and down the shape linearly.
Following are the classes used for animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes con-0 {
0%,
34.99% {
transform: scaley(1);
}
35%,
70% {
transform: scaley(-1);
}
90%,
100% {
transform: scaley(-1) rotate(180deg);
}
}
@keyframes con-1 {
0%,
10%,
70%,
100% {
transform: translateY(-100%) rotate(calc(var(--s, 1) * 135deg));
}
35% {
transform: translateY(0%) rotate(0deg);
}
}
The following way is to declare the CSS Class inside the class block.
.con-triangle {
display: flex;
margin-left: 120px;
width: 120px;
height: 120px;
animation: con-0 1.5s infinite linear;
}
.con-triangle:before,
.con-triangle:after {
content: "";
width: 50%;
background: #f72585;
clip-path: polygon(0 0, 100% 50%, 0% 100%);
animation: inherit;
animation-name: con-1;
transform-origin: bottom left;
}
.con-triangle:before {
clip-path: polygon(0 50%, 100% 0, 100% 100%);
transform-origin: bottom right;
--s: -1;
}
@keyframes con-0 {
0%,
34.99% {
transform: scaley(1);
}
35%,
70% {
transform: scaley(-1);
}
90%,
100% {
transform: scaley(-1) rotate(180deg);
}
}
@keyframes con-1 {
0%,
10%,
70%,
100% {
transform: translateY(-100%) rotate(calc(var(--s, 1) * 135deg));
}
35% {
transform: translateY(0%) rotate(0deg);
}
}
Following is the output for Convertable Triangle Animation animation.
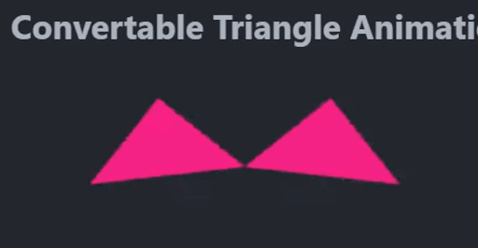
Video Tutorial for convertible triangle in YouTube
You can find the complete coding tutorial in the following YouTube Short video.
Complete Code using HTML and CSS for convertible triangle.
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.con-triangle {
display: flex;
margin-left: 120px;
width: 120px;
height: 120px;
animation: con-0 1.5s infinite linear;
}
.con-triangle:before,
.con-triangle:after {
content: "";
width: 50%;
background: #f72585;
clip-path: polygon(0 0, 100% 50%, 0% 100%);
animation: inherit;
animation-name: con-1;
transform-origin: bottom left;
}
.con-triangle:before {
clip-path: polygon(0 50%, 100% 0, 100% 100%);
transform-origin: bottom right;
--s: -1;
}
@keyframes con-0 {
0%,
34.99% {
transform: scaley(1);
}
35%,
70% {
transform: scaley(-1);
}
90%,
100% {
transform: scaley(-1) rotate(180deg);
}
}
@keyframes con-1 {
0%,
10%,
70%,
100% {
transform: translateY(-100%) rotate(calc(var(--s, 1) * 135deg));
}
35% {
transform: translateY(0%) rotate(0deg);
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Convertable Triangle Animation</h1>
<div class="con-triangle"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Convertible Triangle Animation using CSS and HTML. See you in next tutorial!