Connect with us!
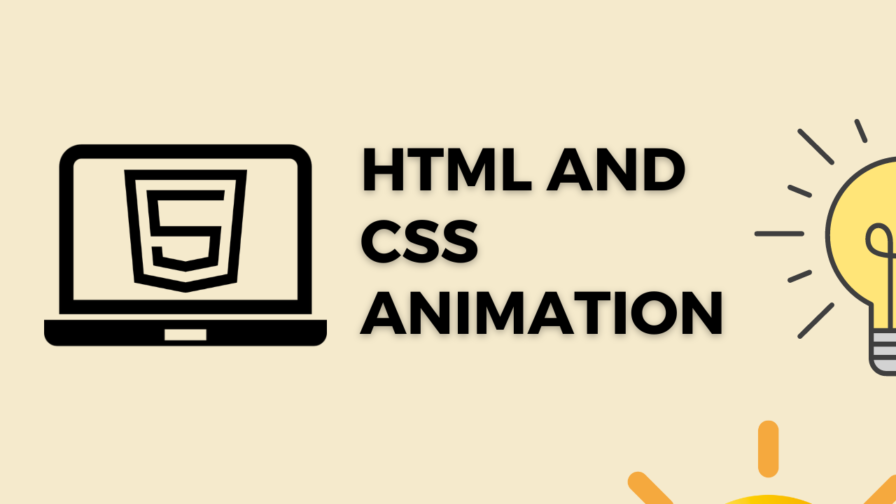
Conveyor Belt Animation using HTML and CSS
Creating Conveyor Belt with CSS animation using HTML and CSS tutorial
Conveyor Belt CSS Properties
Following are the CSS properties used:
Margin-left: The margin-left CSS property sets the margin area on the left side of an element. A positive value places it farther from its neighbors, while a negative value places it closer.
Background-repeat: The background-repeat CSS property sets how background images are repeated. A background image can be repeated along the horizontal and vertical axes, or not repeated at all.
Background: The background shorthand CSS property sets all background style properties at once, such as color, image, origin and size, or repeat method.
Color: The color CSS property sets the foreground color value of an element’s text and text decorations, and sets the currentcolor value. currentcolor may be used as an indirect value on other properties and is the default for other color properties, such as border-color.
Height: The height CSS property specifies the height of an element. By default, the property defines the height of the content area. If box-sizing is set to border-box, however, it instead determines the height of the border area.
Background-size: The background-size CSS property sets the size of the element’s background image. The image can be left to its natural size, stretched, or constrained to fit the available space.
Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.
Conveyor Belt Code Block
Here is the code for implementing the Conveyor Belt Animation.
.con-belt {
width: 270px;
height: 60px;
margin-left: 60px;
color: #f72585;
background: linear-gradient(90deg, currentColor 50%, #0000 0) 0 0%,
linear-gradient(-90deg, currentColor 50%, #0000 0) 50%,
linear-gradient(90deg, currentColor 50%, #0000 0) 0 100%;
background-size: 10px calc(100% / 3);
background-repeat: repeat-x;
}
Yay! We have created the static Conveyor Belt Animation.
Here is the output:
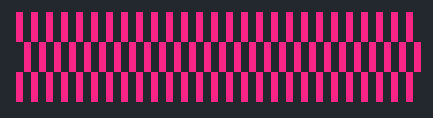
Conveyor Belt CSS Animation
roll animation can be implemented by scaling up and down the shape linearly.
Following are the classes used for animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes roll {
100% {
background-position: -8px 0%, -8px 50%, -8px 100%;
}
}
The following way is to declare the CSS Class inside the class block.
.con-belt {
width: 270px;
height: 60px;
margin-left: 60px;
color: #f72585;
background: linear-gradient(90deg, currentColor 50%, #0000 0) 0 0%,
linear-gradient(-90deg, currentColor 50%, #0000 0) 50%,
linear-gradient(90deg, currentColor 50%, #0000 0) 0 100%;
background-size: 10px calc(100% / 3);
background-repeat: repeat-x;
animation: roll 0.25s infinite linear;
}
@keyframes roll {
100% {
background-position: -8px 0%, -8px 50%, -8px 100%;
}
}
Following is the output for Conveyor Belt Animation animation.
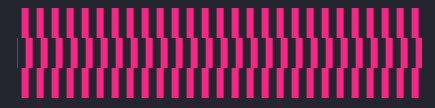
Conveyor Belt Video Tutorial
You can find the complete coding tutorial in the following YouTube Short video.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.con-belt {
width: 270px;
height: 60px;
margin-left: 60px;
color: #f72585;
background: linear-gradient(90deg, currentColor 50%, #0000 0) 0 0%,
linear-gradient(-90deg, currentColor 50%, #0000 0) 50%,
linear-gradient(90deg, currentColor 50%, #0000 0) 0 100%;
background-size: 10px calc(100% / 3);
background-repeat: repeat-x;
animation: roll 0.25s infinite linear;
}
@keyframes roll {
100% {
background-position: -8px 0%, -8px 50%, -8px 100%;
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Conveyor Belt - Salow Studios</h1>
<div class="con-belt"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Conveyor Belt Animation Animation using CSS and HTML. See you in next tutorial!