Connect with us!
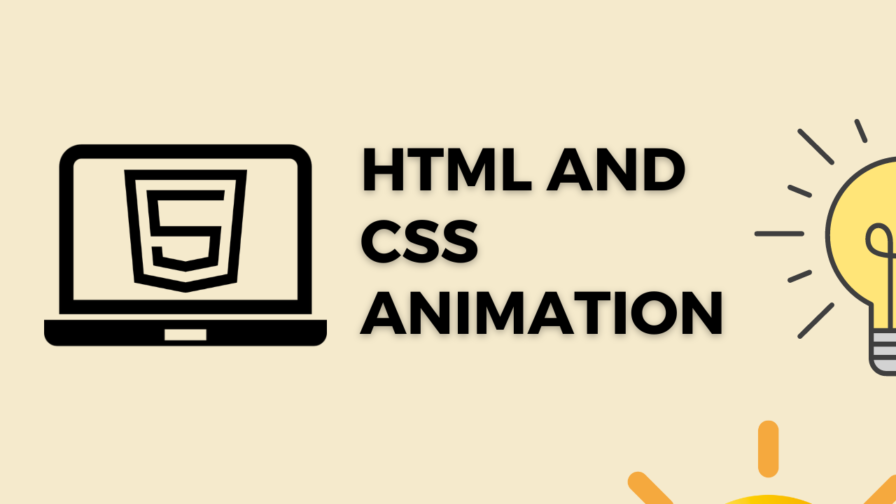
Dancing dots Animation using CSS coding
Loding screen animation with dancing dots using HTML and CSS.
Loading screen is done by pure CSS Animation.
CSS Properties
Following are the CSS properties used:
- Background: The background shorthand CSS property sets all background style properties at once, such as color, image, origin and size, or repeat method.
- Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.
- Background-size: The background-size CSS property sets the size of the element’s background image. The image can be left to its natural size, stretched, or constrained to fit the available space.
- Aspect-ratio: The aspect-ratio CSS property sets a preferred aspect ratio for the box, which will be used in the calculation of auto sizes and some other layout functions.
Dancing dots Code Block
Here is the code for implementing the Dancing dots Animation.
.dancing-dots {
width: 240px;
aspect-ratio: 2;
--_g: no-repeat
radial-gradient(farthest-side, rgb(246, 27, 27) 90%, #0000);
background: var(--_g) 0 50%, var(--_g) 50% 50%, var(--_g) 50% 50%,
var(--_g) 100% 50%;
background-size: 25% 50%;
}
Yay! We have created the static Dancing dots Animation.
Here is the output:
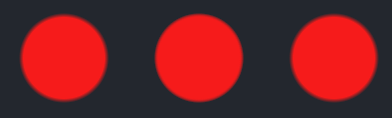
Dancing dots CSS Animation
slide animation can be implemented by scaling up and down the shape linearly.
Following are the classes used for animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes slide {
33% {
background-position: 0 0, 50% 100%, 50% 100%, 100% 0;
}
66% {
background-position: 50% 0, 0 100%, 100% 100%, 50% 0;
}
100% {
background-position: 50% 50%, 0 50%, 100% 50%, 50% 50%;
}
}
The following way is to declare the CSS Class inside the class block.
.dancing-dots {
width: 240px;
aspect-ratio: 2;
--_g: no-repeat
radial-gradient(farthest-side, rgb(246, 27, 27) 90%, #0000);
background: var(--_g) 0 50%, var(--_g) 50% 50%, var(--_g) 50% 50%,
var(--_g) 100% 50%;
background-size: 25% 50%;
animation: slide 1s infinite linear;
}
@keyframes slide {
33% {
background-position: 0 0, 50% 100%, 50% 100%, 100% 0;
}
66% {
background-position: 50% 0, 0 100%, 100% 100%, 50% 0;
}
100% {
background-position: 50% 50%, 0 50%, 100% 50%, 50% 50%;
}
}
Following is the output for Dancing dots Animation animation.
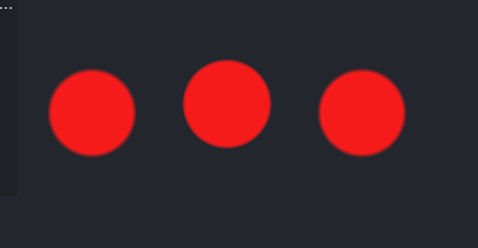
Dancing dots Video Tutorial
You can find the complete coding tutorial in the following YouTube Short video.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.dancing-dots {
width: 240px;
aspect-ratio: 2;
--_g: no-repeat
radial-gradient(farthest-side, rgb(246, 27, 27) 90%, #0000);
background: var(--_g) 0 50%, var(--_g) 50% 50%, var(--_g) 50% 50%,
var(--_g) 100% 50%;
background-size: 25% 50%;
animation: slide 1s infinite linear;
}
@keyframes slide {
33% {
background-position: 0 0, 50% 100%, 50% 100%, 100% 0;
}
66% {
background-position: 50% 0, 0 100%, 100% 100%, 50% 0;
}
100% {
background-position: 50% 50%, 0 50%, 100% 50%, 50% 50%;
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Dancing dots Animation</h1>
<div class="dancing-dots"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Dancing dots Animation Animation using CSS and HTML. See you in next tutorial!