Connect with us!
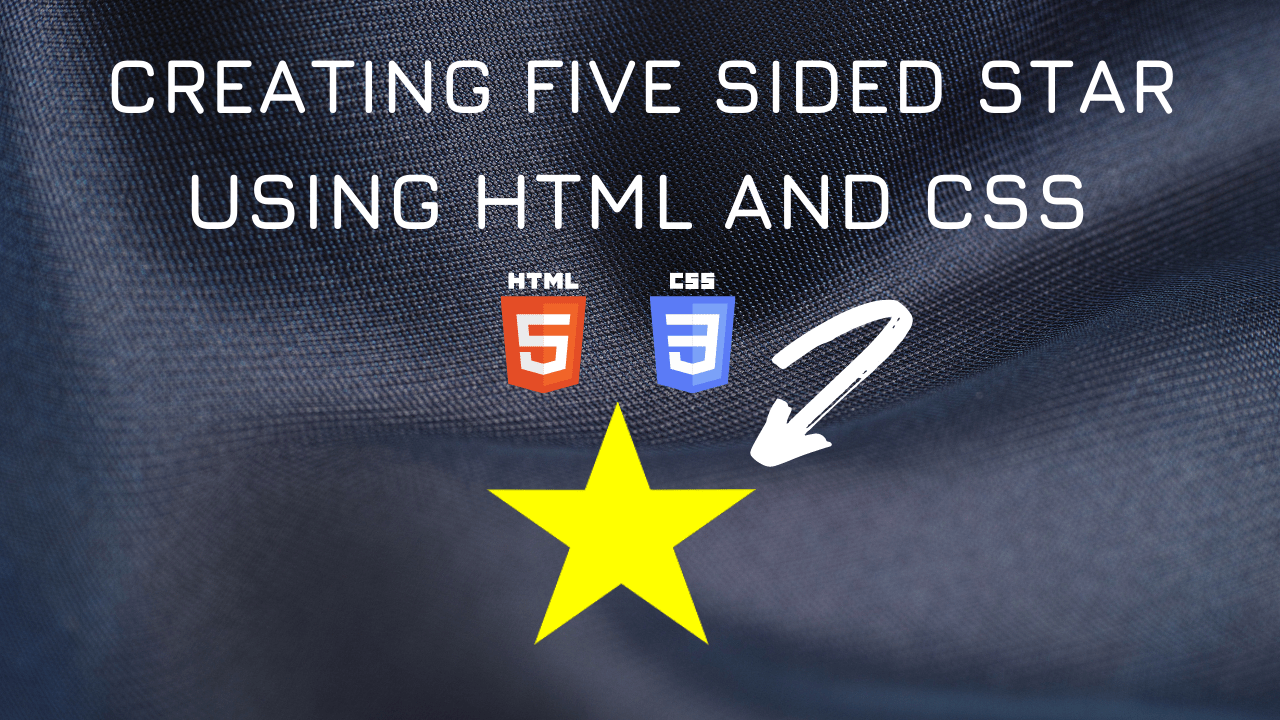
Creating Five Sided Star for rating using HTML and CSS
One of the best way to get feedback from a website is by providing rating system. Have you wondered how to create a star using CSS? In this article, we will see how to create a Five sided star using HTML and CSS in Visual Studio Code.
Here is the code for creating the Five Sided Star.
The border-right property sets the style of an element’s right border.
The border-bottom property sets the style of an element’s bottom border.
The border-left property sets the style of an element’s left border.
The transform property applies a 2D or 3D transformation to an element. This property allows you to rotate, scale, move, skew, etc., elements.
Table of Contents
Code Block
Here is the CSS class code:
.star-five {
margin: 70px 0;
position: relative;
display: block;
color: yellow;
width: 0px;
height: 0px;
border-right: 100px solid transparent;
border-bottom: 70px solid yellow;
border-left: 100px solid transparent;
transform: rotate(35deg);
}
.star-five:before {
border-bottom: 80px solid yellow;
border-left: 30px solid transparent;
border-right: 30px solid transparent;
position: absolute;
height: 0;
width: 0;
top: -45px;
left: -65px;
display: block;
content: '';
transform: rotate(-35deg);
}
.star-five:after {
position: absolute;
display: block;
color: yellow;
top: 3px;
left: -105px;
width: 0px;
height: 0px;
border-right: 100px solid transparent;
border-bottom: 70px solid yellow;
border-left: 100px solid transparent;
transform: rotate(-70deg);
content: '';
}
The result will be as shown below:
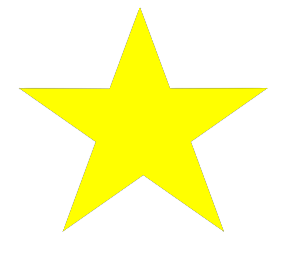
You can find the complete coding tutorial in the following Youtube video.
Video Tutorial for Creating Star
Here is the complete HTML and CSS code
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.star-five {
margin: 70px 0;
position: relative;
display: block;
color: yellow;
width: 0px;
height: 0px;
border-right: 100px solid transparent;
border-bottom: 70px solid yellow;
border-left: 100px solid transparent;
transform: rotate(35deg);
}
.star-five:before {
border-bottom: 80px solid yellow;
border-left: 30px solid transparent;
border-right: 30px solid transparent;
position: absolute;
height: 0;
width: 0;
top: -45px;
left: -65px;
display: block;
content: '';
transform: rotate(-35deg);
}
.star-five:after {
position: absolute;
display: block;
color: yellow;
top: 3px;
left: -105px;
width: 0px;
height: 0px;
border-right: 100px solid transparent;
border-bottom: 70px solid yellow;
border-left: 100px solid transparent;
transform: rotate(-70deg);
content: '';
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Five Sided Star</h1>
<div class="star-five"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own five sided star for rating in your app or any project you do. Good luck and happy coding !