Connect with us!
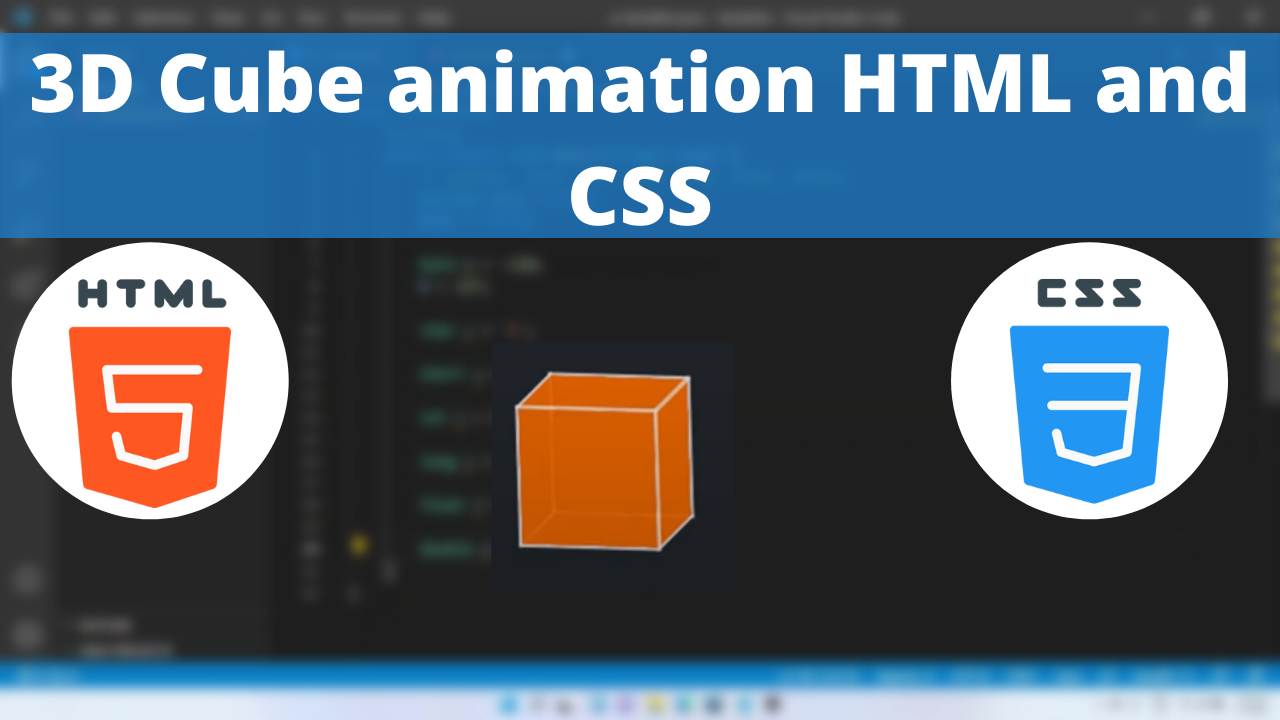
3D Cube animation HTML and CSS tutorial for beginners
Cube is a regular solid with six square faces. Square consists of the length and width of same size. Have you ever wondered how to create them using CSS and use it in your website? In tis tutorial we will be looking into how to design 3D Cube spin loader animation.
The most important properties that we use to create the satellite with spin Animation are:
The transform
CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
The animation
shorthand CSS property applies an animation between styles. It is a shorthand for animation-name
, animation-duration
, animation-timing-function
, animation-delay
, animation-iteration-count
, animation-direction
, animation-fill-mode
, and animation-play-state
.
Table of Contents
3D Cube animation Code Block
Here is the code for implementing 3D Cube.
.cube {
width: 20px;
height: 20px;
transform-style: preserve-3d;
margin: 50px;
}
.face {
width: 100px;
height: 100px;
background: #ff6d00;
border: 2px solid white;
position: absolute;
opacity: 0.75;
transform: translateZ(50px);
}
.front {
transform: translateZ(50px);
}
.back {
transform: translateZ(-50px) rotateY(180deg);
}
.left {
transform: translateX(-50px) rotateY(-90deg);
}
.right {
transform: translateX(50px) rotateY(90deg);
}
.top {
transform: translateY(-50px) rotateX(90deg);
}
.bottom {
transform: translateY(50px) rotateX(-90deg);
}
Yay! We have created the static 3D Cube.
Here is the output:
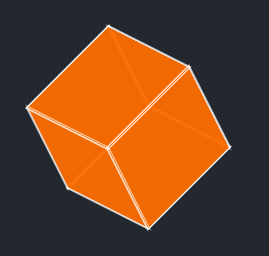
3D Cube animation CSS Animation
3D Cube spin is a tricky animation. It is achieved by rotating the object at the bottom of first object.
Following are the classes used for animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes turn {
from {
transform: rotate3d(0, 0, 0, 0);
}
to {
transform: rotate3d(1, 1, 0, 360deg);
}
}
The following way is to declare the CSS Class inside the class block.
.cube {
width: 20px;
height: 20px;
transform-style: preserve-3d;
animation: turn 5s linear infinite;
margin: 50px;
}
.face {
width: 100px;
height: 100px;
background: #ff6d00;
border: 2px solid white;
position: absolute;
opacity: 0.75;
transform: translateZ(50px);
}
.front {
transform: translateZ(50px);
}
.back {
transform: translateZ(-50px) rotateY(180deg);
}
.left {
transform: translateX(-50px) rotateY(-90deg);
}
.right {
transform: translateX(50px) rotateY(90deg);
}
.top {
transform: translateY(-50px) rotateX(90deg);
}
.bottom {
transform: translateY(50px) rotateX(-90deg);
}
Following is the output of 3D Cube spin animation
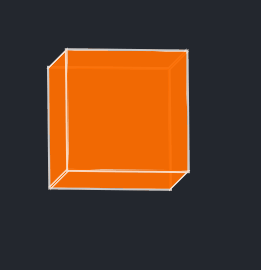
3D Cube animation Video Tutorial
You can find the complete coding tutorial in the following YouTube video.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
@keyframes turn {
from {
transform: rotate3d(0, 0, 0, 0);
}
to {
transform: rotate3d(1, 1, 0, 360deg);
}
}
.cube {
width: 20px;
height: 20px;
transform-style: preserve-3d;
animation: turn 5s linear infinite;
margin: 50px;
}
.face {
width: 100px;
height: 100px;
background: #ff6d00;
border: 2px solid white;
position: absolute;
opacity: 0.75;
transform: translateZ(50px);
}
.front {
transform: translateZ(50px);
}
.back {
transform: translateZ(-50px) rotateY(180deg);
}
.left {
transform: translateX(-50px) rotateY(-90deg);
}
.right {
transform: translateX(50px) rotateY(90deg);
}
.top {
transform: translateY(-50px) rotateX(90deg);
}
.bottom {
transform: translateY(50px) rotateX(-90deg);
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>3D Cube Animation</h1>
<div class="cube">
<div class="face top"></div>
<div class="face bottom"></div>
<div class="face left"></div>
<div class="face right"></div>
<div class="face front"></div>
<div class="face back"></div>
</div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own 3D cube Spin Animation using CSS and HTML. Good luck and happy coding !