Connect with us!
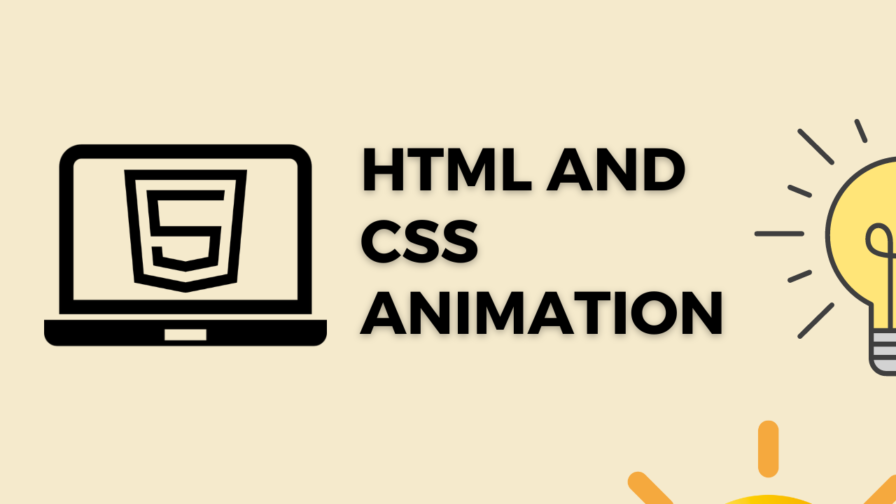
Globe Animation using HTML and CSS
A globe is a spherical model of Earth, of some other celestial body, or of the celestial sphere. Globes serve purposes similar to maps, but unlike maps, they do not distort the surface that they portray except to scale it down. A model globe of Earth is called a terrestrial globe. The CSS properties used are as follows..
CSS Properties
Following are the CSS properties used:
Background: The background shorthand CSS property sets all background style properties at once, such as color, image, origin and size, or repeat method. Component properties not set in the background shorthand property value declaration are set to their default values.”/><style media=”print
Margin-left: The margin-left CSS property sets the margin area on the left side of an element. A positive value places it farther from its neighbors, while a negative value places it closer.”/><style media=”print
Border-radius: The border-radius CSS property rounds the corners of an element’s outer border edge. You can set a single radius to make circular corners, or two radii to make elliptical corners.”/><style media=”print
-webkit-mask: The mask CSS shorthand property hides an element (partially or fully) by masking or clipping the image at specific points.”/><style media=”print
Width: The width CSS property sets an element’s width. By default, it sets the width of the content area, but if box-sizing is set to border-box, it sets the width of the border area.”/><style media=”print
Height: The height CSS property specifies the height of an element. By default, the property defines the height of the content area. If box-sizing is set to border-box, however, it instead determines the height of the border area.”/><style media=”print
Mask-composite: The mask-composite CSS property represents a compositing operation used on the current mask layer with the mask layers below it.”/><style media=”print
-webkit-mask-composite: The -webkit-mask-composite property specifies the manner in which multiple mask images applied to the same element are composited with one another. Mask images are composited in the opposite order that they are declared with the -webkit-mask-image property.”/><style media=”print
-webkit-mask-repeat: The mask-repeat CSS property sets how mask images are repeated. A mask image can be repeated along the horizontal axis, the vertical axis, both axes, or not repeated at all.”/><style media=”print
Globe Animation CSS Code Block
Here is the code for implementing the Globe Animation.
.globe {
width: 70px;
height: 70px;
margin-left: 70px;
background: #ffd600;
border-radius: 50px;
-webkit-mask: radial-gradient(
circle 31px at 50% calc(100% + 13px),
#000 95%,
#0000
)
top 4px left 50%,
radial-gradient(circle 31px, #000 95%, #0000) center,
radial-gradient(circle 31px at 50% -13px, #000 95%, #0000) bottom 4px
left 50%,
linear-gradient(#000 0 0);
-webkit-mask-composite: xor;
mask-composite: exclude;
-webkit-mask-repeat: no-repeat;
}
Yay! We have created the static Globe Animation.
Here is the output:
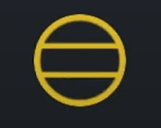
Globe CSS Animation
in-out animation can be implemented by scaling up and down the shape linearly.
Following are the classes used for animation.
The @keyframes CSS at-rule controls the intermediate steps in a CSS animation sequence by defining styles for keyframes (or waypoints) along the animation sequence. This gives more control over the intermediate steps of the animation sequence than transitions.
The transform CSS property lets you rotate, scale, skew, or translate an element. It modifies the coordinate space of the CSS visual formatting model.
Here is the CSS animation class implementing CSS.
@keyframes in-out {
0% {
-webkit-mask-size: 0 18px, 0 18px, 0 18px, auto;
}
16.67% {
-webkit-mask-size: 100% 18px, 0 18px, 0 18px, auto;
}
33.33% {
-webkit-mask-size: 100% 18px, 100% 18px, 0 18px, auto;
}
50% {
-webkit-mask-size: 100% 18px, 100% 18px, 100% 18px, auto;
}
66.67% {
-webkit-mask-size: 0 18px, 100% 18px, 100% 18px, auto;
}
83.33% {
-webkit-mask-size: 0 18px, 0 18px, 100% 18px, auto;
}
100% {
-webkit-mask-size: 0 18px, 0 18px, 0 18px, auto;
}
}
The following way is to declare the CSS Class inside the class block.
.globe {
width: 70px;
height: 70px;
margin-left: 70px;
background: #ffd600;
border-radius: 50px;
-webkit-mask: radial-gradient(
circle 31px at 50% calc(100% + 13px),
#000 95%,
#0000
)
top 4px left 50%,
radial-gradient(circle 31px, #000 95%, #0000) center,
radial-gradient(circle 31px at 50% -13px, #000 95%, #0000) bottom 4px
left 50%,
linear-gradient(#000 0 0);
-webkit-mask-composite: xor;
mask-composite: exclude;
-webkit-mask-repeat: no-repeat;
animation: in-out 1.5s infinite;
}
@keyframes in-out {
0% {
-webkit-mask-size: 0 18px, 0 18px, 0 18px, auto;
}
16.67% {
-webkit-mask-size: 100% 18px, 0 18px, 0 18px, auto;
}
33.33% {
-webkit-mask-size: 100% 18px, 100% 18px, 0 18px, auto;
}
50% {
-webkit-mask-size: 100% 18px, 100% 18px, 100% 18px, auto;
}
66.67% {
-webkit-mask-size: 0 18px, 100% 18px, 100% 18px, auto;
}
83.33% {
-webkit-mask-size: 0 18px, 0 18px, 100% 18px, auto;
}
100% {
-webkit-mask-size: 0 18px, 0 18px, 0 18px, auto;
}
}
Following is the output for Globe Animation animation.
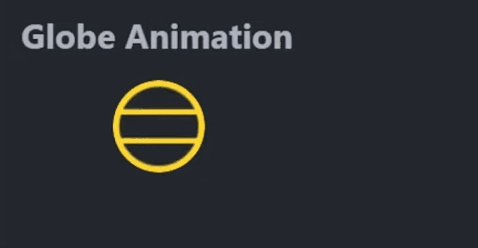
Globe CSS Video Tutorial
You can find the complete coding tutorial in the following YouTube Short video.
Complete Code using HTML and CSS
Copy and paste the complete code in Visual studio code to view the output.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.globe {
width: 70px;
height: 70px;
margin-left: 70px;
background: #ffd600;
border-radius: 50px;
-webkit-mask: radial-gradient(
circle 31px at 50% calc(100% + 13px),
#000 95%,
#0000
)
top 4px left 50%,
radial-gradient(circle 31px, #000 95%, #0000) center,
radial-gradient(circle 31px at 50% -13px, #000 95%, #0000) bottom 4px
left 50%,
linear-gradient(#000 0 0);
-webkit-mask-composite: xor;
mask-composite: exclude;
-webkit-mask-repeat: no-repeat;
animation: in-out 1.5s infinite;
}
@keyframes in-out {
0% {
-webkit-mask-size: 0 18px, 0 18px, 0 18px, auto;
}
16.67% {
-webkit-mask-size: 100% 18px, 0 18px, 0 18px, auto;
}
33.33% {
-webkit-mask-size: 100% 18px, 100% 18px, 0 18px, auto;
}
50% {
-webkit-mask-size: 100% 18px, 100% 18px, 100% 18px, auto;
}
66.67% {
-webkit-mask-size: 0 18px, 100% 18px, 100% 18px, auto;
}
83.33% {
-webkit-mask-size: 0 18px, 0 18px, 100% 18px, auto;
}
100% {
-webkit-mask-size: 0 18px, 0 18px, 0 18px, auto;
}
}
</style>
<title>Salow Studios</title>
</head>
<body>
<h1>Globe Animation</h1>
<div class="globe"></div>
</body>
</html>
Thank you for reading this article. I hope that it helps you creating your own Globe Animation Animation using CSS and HTML. See you in next tutorial!