Connect with us!
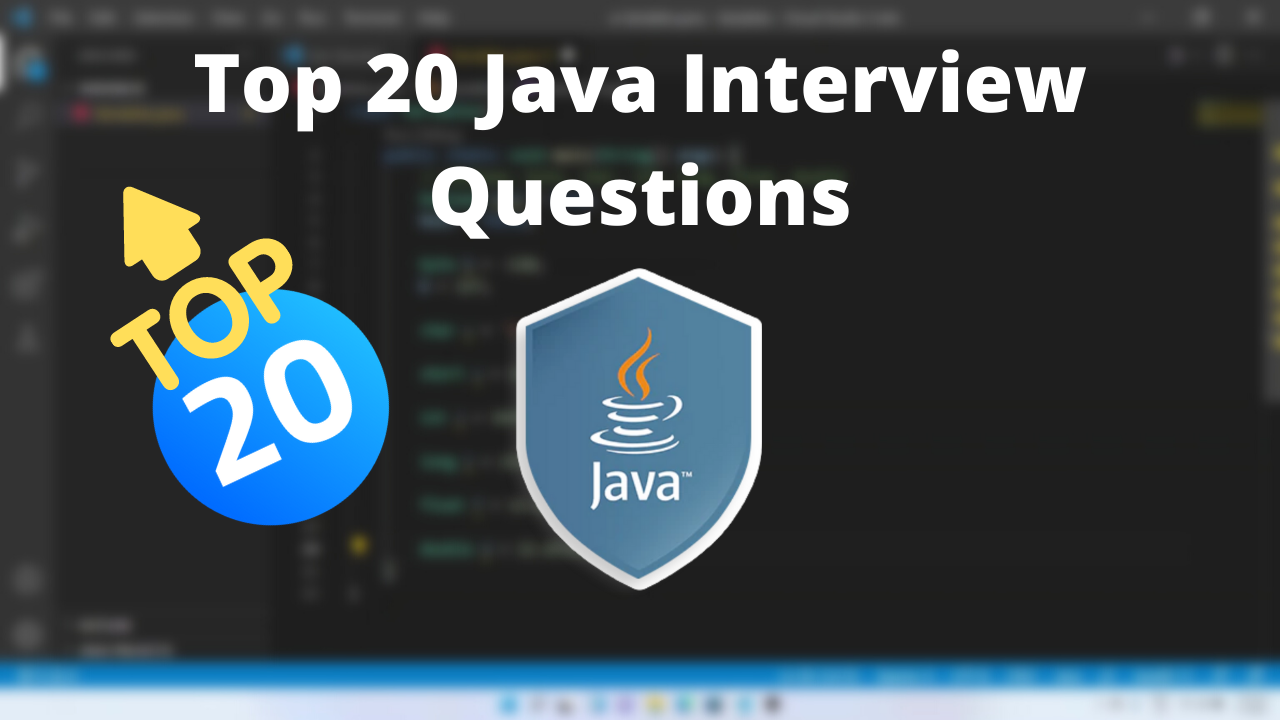
Top 20 Java Interview Questions
In this article we will look into three topics. First one is most commonly asked interview questions for java developer position. Secondly, theoretical answers with examples. Finally, some tips for how you can standout during your interview and ace it.
Table of Contents
1. What is the meaning of the term “platform independent” and how can it be related to JAVA?
The term “platform independent” means that a programming language is dependent on any one platform to run. After the code is complied, it can be executed on any platform or operating system.
In JAVA the complier will convert code to byte code and this byte code can run on any operating system using Java virtual machine (JVM).
2. What is difference between JDK,JRE and JVM?
JVM ( Java Virtual Machine) is an abstract machine. It provides a runtime environment in which java bytecode is executed.
JRE(Java Runtime Environment) is a runtime environment which implements JVM and provides all class libraries and other files that JVM uses at runtime.
JDK( Java Development Kit) is the tool necessary to compile, document and package java programs, The JDK includes JRE.
3. Why pointers are not used in Java?
JVM is responsible for implicit memory allocation, thus in order to avoid direct access to memory by the user, pointers are not used in JAVA.
4. What is Just in Time complier in Java?
When we complie the java file the complier produces the dot class file which consists of byte code. The byte code is verified but the byte code verifier in JVM. The execution engine will convert the byte code into machine code and this is called just in time compiling. JIT complies code to machine level directly for higher speed code execution.
5. What is the default value stored in Local variables?
Neither the local variables nor any primitives and object references have any default values stored in them. So the local variables do not have any default values which means that they can be declared and assigned a value before the variables are used for the first time or else, the complier throws an error saying “variable might not have been initialised“.
6. Differentiate between instance and local variable
Instance variables are declared inside a class and the scope is limited to only a specific object.
Local variables can be declared anywhere inside a method, or a specific block of code. Also, the scope is limited to block of code where the variable is declared.
7. What happens when main() is not declared as static?
When main method is not declared as static, then the program may be complied perfectly, but it ends up with an ambiguity and throws a run time error that reads “NoSuchMethodError”.
8. Why strings are immutable in Java?
- String pool is a place where all the literals of string are stored and this requires string to be immutable or else the shared reference can be changed from anywhere.
- the next main reason is due to security in java makes string immutable hence no one can change reference of string once created.
9. Is string a datatype in Java?
String is not a primitive data type in Java. When a string is created in java, it is an object of Java.Lang.String class that gets created. After creation of the string object , all built-in methods of the string class can be used on the string object.
10 . How can you make a class immutable?
- Declare the class as final so it can’t be extended
- Make all fields private so that direct access is not allowed
- Make class mutable fields final hence the values can be assigned only once
- Initialise all the fields using constructor
- Do not provide setter methods for variables
11. What is overriding in Java?
In OOP language, overriding is a feature which allows a subclass or child class to provide specific implementation of a method that is already provided by one of its super classes or parent classes. When a method in a subclass has the same name or parameter and return type as a method in its super-class, then the method in the subclass is said to override the method in the superclass.
12. Can we override a private or static method in Java?
- We cannot override a private or static method in Java
- We cannot override a private method in sub class because it’s not accessible, what we can do is create another private method with the same name in the child class.
- for static methods if you create a similar method with same return super and same method arguments in child class then it will hide the superclass method, this is knows as method hiding.
13. What is “Wrapper class” in Java ?
“Wrapper class” in Java is to create objects. Essentially, the tell Java primitives to change into reference types called objects.
The reason it gets the name “wrapper class” is each java’s eight data types has a class dedicated to it. These classes wrap the primitive data type into a n object of that class.
14. What is the difference between Public, Private, Default and Protected?
- The members of a class that are preceded with the public modifier are accessible by the classes present within the package or outside the package.
- Private allows us to hide member variables and method from other classed, these are accessible only inside the class and cannot be altered by any methods outside the class.
- Default is also similar to public modifier, if you don’t specify any other modifiers the scope of the data members and methods is default and can be accessed by the classes present within the package.
- Protected modifier allows the members of the classes to be accessible to all the other classes within the package, subclass or outside the package. The protected modifier becomes important while implementing inheritance.
15. What is the difference between the keywords final, finally and finalize ?
- Final is used to apply restrictions on class, method and variable. Final class can’t be inherited , final method can’t be overridden and final variable value can’t be changed. In the below example the
variable "a"
is declared as final.
class finalExample{
public static void main(String args[]){
final int a = 100;
a = 500;
}
}
- Finally is used to place important code, it will be executed whether exception is handled or not
class finallyExample{
public static void main(String args[]){
try {
int x =100;
}catch{Exception e }{System.out.println(e);}
finally
{System.out.println("finally block is executed");}
}
}
- Finalize is used to perform clean up processing just before object is garbage collected.
class finalizeExample{
public void finalize(){
System.out.println("Finalize is called");
}
public static void main(String args[])
{
finalizeExample f1 = new finalizeExample();
finalizeExample f2 = new finalizeExample();
f1 = NULL;
f2 = NULL;
system.gc();
}
}
16. Does “finally” always execute in java?
Finally does not execute in the following cases
- “System.exit()” function
- whenever system crashes
17. What is Singleton class in Java ?
In java, we can make the constructor of a class as private, then that particular class can generate only a single object. This type of class is know as a Singleton Class.Singleton classes are helpful for the situations when there is a need to limit the number of objects for a class.
The apt example of singleton usage is when we have limited or only one connection to a database because of any licensing issues or driver limitations.
18. What is Exception in java? How to handle it?
In Java, an exception is an event that disrupts the normal flow of the program. Exception handing is a mechanism in which we handle runtime errors such as ClassNotFoundException, IOException, SQLException and RemoteException etc. Below is an example for execution handling in java using try-catch statement.
public class JavaExceptionExample{
public static void main(String args[]){
try{
//code that may raise exception
int data=500/0;
}catch(ArithmeticException e){System.out.println(e);}
//rest code of the program
System.out.println("rest of the code...");
}
}
19. Is it necessary for a try block to be followed by a catch block in Exception handling?
Try block needs to be followed by either Catch block or Finally block or both. Any exception thrown from try block needs to be either caught in the catch block or else any specific tasks to be performed before code abortion are put in the finally block.
20. How garbage collection is done in java?
In java, when an object is not referenced any more, garbage collection takes places and the object is destroyed automatically. For automatic garbage collection java calls either System.gc() or Runtime.gc() method.
Tips for Interview
In order to prepare an interview do research in four areas as given below:
- Prepare a summary of your relevant qualifications for the position your are applying for, how you can meet the employers needs and how you can add value to them.
- Get a clear understanding of the position.
- Get to know about the employer – eg: the common question an employer can ask is why you want to work for us , what do you know about us.
- Prepare a very good introduction about yourself to create a good first impressions about you.